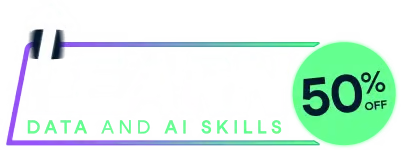
Last chance! 50% off unlimited learning
Sale ends in
As in try
, the result of an expression if it works.
If it fails, execution is not halted, but an invisible try-error class object is
returned and (unless silent=TRUE) a message catted
to the console.
Unlike try
, tryStack
also returns the calling stack to
trace errors and warnings and ease debugging.
tryStack(expr, silent = FALSE, warn = TRUE, short = TRUE,
file = "", removetry = TRUE, skip = NULL)
Expression to try, potentially wrapped in curly braces if spanning several commands.
Logical: Should printing of error message + stack be suppressed? Does not affect warnings and messages. DEFAULT: FALSE
Logical: should trace be abbreviated to upper -> middle -> lower? If NA, it is set to TRUE for warnings and messages, FALSE for errors. DEFAULT: TRUE
File name passed to cat
.
If given, Errors will be appended to the file after two empty lines.
if warn=T
and file!="", warnings and messages will not be shown,
but also appended to the file.
This is useful in lapply simulation runs.
DEFAULT: "" (catted to the console)
Logical: should all stack entries matching typical tryCatch
expressions be removed? Unless the call contains customized
tryCatch
code, this can be left to the DEFAULT: TRUE
Character string(s) to be removed from the stack. e.g. "eval(expr, p)". Use short=F to find exact matches. DEFAULT: NULL
Value of expr
if evaluated successfully. If not, an invisible
object of class "try-error" as in try
with the stack in object[2]
.
For nested tryStack calls, object[3], object[4]
etc. will contain "-- empty error stack --"
try
, traceCall
,
http://r.789695.n4.nabble.com/Stack-trace-td4021537.html,
http://stackoverflow.com/questions/15282471/get-stack-trace-on-trycatched-error-in-r,
http://stackoverflow.com/questions/1975110/printing-stack-trace-and-continuing-after-error-occurs-in-r,
http://stackoverflow.com/questions/16879821/save-traceback-on-error-using-trycatch
# NOT RUN {
# Functions -----
lower <- function(a) {message("fake message, a = ", a); a+10}
middle <- function(b) {plot(b, main=b) ; warning("fake warning, b = ", b); lower(b) }
upper <- function(c) {cat("printing c:", c, "\n") ; middle(c)}
d <- upper(42)
d
rm(d)
# Classical error management with try -----
is.error( d <- upper("42"), TRUE, TRUE) # error, no d creation
traceback() # calling stack, but only in interactive mode
d <- try(upper("42"), silent=TRUE) # d created
cat(d) # with error message, but no traceback
inherits(d, "try-error") # use for coding
# way cooler with tryStack -----
d <- tryStack(upper("42") ) # like try, but with traceback, even for warnings
cat(d)
d <- tryStack(upper("42"), silent=TRUE, warn=0) # don't trace warnings
d <- tryStack(upper("42"), short=FALSE)
tryStack(upper(42)) # returns normal output, but warnings are easier to debug
# Note: you can also set options(showWarnCalls=TRUE)
stopifnot(inherits(d, "try-error"))
stopifnot(tryStack(upper(42))==52)
# }
# NOT RUN {
## file writing not wanted by CRAN checks
d <- tryStack(upper("42"), silent=TRUE, file="log.txt")
openFile("log.txt")
unlink("log.txt")
# }
# NOT RUN {
op <- options(warn=2)
d <- try(upper("42") )
cat(d)
d <- tryStack(upper("42") )
d <- tryStack(upper("42"), warn=FALSE)
cat(d)
options(op) ; rm(op)
# Nested calls -----
f <- function(k) tryStack(upper(k), silent=TRUE)
d <- f(42) ; cat("-----\n", d, "\n-----\n") ; rm(d)
d <- f("42") ; cat("-----\n", d, "\n-----\n") ; rm(d)
d <- tryStack(f(4) ) ; cat("-----\n", d, "\n-----\n") ; rm(d)
# warnings in nested calls are printed twice, unless warn=0
d <- tryStack(f(4), warn=0) # could also be set within 'f'
d <- tryStack(f("4")) ; cat("-----\n", d, "\n-----\n")
d[1:3] ; rm(d)
# empty stack at begin - because of tryStack in f, no real error happened in f
# Other tests -----
cat( tryStack(upper("42")) )
f <- function(k) tryStack(stop("oh oh"))
d <- f(42) ; cat("-----\n", d, "\n-----\n") ; rm(d) # level 4 not helpful, but OK
# stuff with base::try
f <- function(k) try(upper(k), silent=TRUE)
d <- f(42) ; cat("-----\n", d, "\n-----\n") ; rm(d)
d <- f("42") ; cat("-----\n", d, "\n-----\n") ; rm(d) # regular try output
f2 <- function(k) tryStack(f(k), warn=0, silent=TRUE)
d <- f2(42) ; cat("-----\n", d, "\n-----\n") ; rm(d)
d <- f2("42") ; cat("-----\n", d, "\n-----\n") ; rm(d) # try -> no error.
# -> Use tryCatch and you can nest those calls. note that d gets longer.
# }
Run the code above in your browser using DataLab