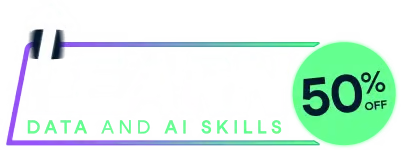
Last chance! 50% off unlimited learning
Sale ends in
Launch a popup to ask confirmation to the user
confirmSweetAlert(session, inputId, title = NULL, text = NULL,
type = "question", btn_labels = c("Cancel", "Confirm"),
btn_colors = NULL, closeOnClickOutside = FALSE,
showCloseButton = FALSE, html = FALSE, ...)
The session
object passed to function given to shinyServer.
The input
slot that will be used to access the value.
If in a Shiny module, it use same logic than inputs : use namespace in UI, not in server.
Title of the alert.
Text of the alert, can contains HTML tags.
Type of the alert : info, success, warning or error.
Labels for buttons, cancel button (FALSE
) first then confirm button (TRUE
).
Colors for buttons.
Decide whether the user should be able to dismiss the modal by clicking outside of it, or not.
Show close button in top right corner of the modal.
Does text
contains HTML tags ?
Additional arguments (not used)
# NOT RUN {
# }
# NOT RUN {
if (interactive()) {
library("shiny")
library("shinyWidgets")
ui <- fluidPage(
tags$h1("Confirm sweet alert"),
actionButton(
inputId = "launch",
label = "Launch confirmation dialog"
),
verbatimTextOutput(outputId = "res"),
uiOutput(outputId = "count")
)
server <- function(input, output, session) {
# Launch sweet alert confirmation
observeEvent(input$launch, {
confirmSweetAlert(
session = session,
inputId = "myconfirmation",
title = "Want to confirm ?"
)
})
# raw output
output$res <- renderPrint(input$myconfirmation)
# count click
true <- reactiveVal(0)
false <- reactiveVal(0)
observeEvent(input$myconfirmation, {
if (isTRUE(input$myconfirmation)) {
x <- true() + 1
true(x)
} else {
x <- false() + 1
false(x)
}
}, ignoreNULL = TRUE)
output$count <- renderUI({
tags$span(
"Confirm:", tags$b(true()),
tags$br(),
"Cancel:", tags$b(false())
)
})
}
shinyApp(ui, server)
# other options :
ui <- fluidPage(
tags$h1("Confirm sweet alert"),
actionButton(
inputId = "launch1",
label = "Launch confirmation dialog"
),
verbatimTextOutput(outputId = "res1"),
tags$br(),
actionButton(
inputId = "launch2",
label = "Launch confirmation dialog (with normal mode)"
),
verbatimTextOutput(outputId = "res2"),
tags$br(),
actionButton(
inputId = "launch3",
label = "Launch confirmation dialog (with HTML)"
),
verbatimTextOutput(outputId = "res3")
)
server <- function(input, output, session) {
observeEvent(input$launch1, {
confirmSweetAlert(
session = session,
inputId = "myconfirmation1",
type = "warning",
title = "Want to confirm ?"
)
})
output$res1 <- renderPrint(input$myconfirmation1)
observeEvent(input$launch2, {
confirmSweetAlert(
session = session,
inputId = "myconfirmation2",
type = "warning",
title = "Are you sure ??",
btn_labels = c("Nope", "Yep"),
btn_colors = c("#FE642E", "#04B404")
)
})
output$res2 <- renderPrint(input$myconfirmation2)
observeEvent(input$launch3, {
confirmSweetAlert(
session = session,
inputId = "myconfirmation3",
title = NULL,
text = tags$b(
icon("file"),
"Do you really want to delete this file ?",
style = "color: #FA5858;"
),
btn_labels = c("Cancel", "Delete file"),
btn_colors = c("#00BFFF", "#FE2E2E"),
html = TRUE
)
})
output$res3 <- renderPrint(input$myconfirmation3)
}
shinyApp(ui = ui, server = server)
}
# }
Run the code above in your browser using DataLab