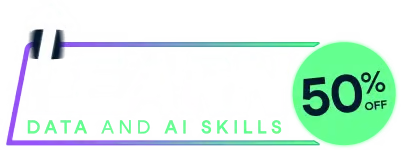
Last chance! 50% off unlimited learning
Sale ends in
Calculate one or multiple bootstrap confidence intervals, given a sample of bootstrap replications.
bootstrap_CIs(
boot_est,
boot_se = NULL,
est = NULL,
se = NULL,
influence = NULL,
CI_type = "percentile",
level = 0.95,
B_vals = length(boot_est),
reps = 1L,
format = "wide",
seed = NULL
)
If format = "wide"
, the function returns a data.frame
with reps
rows per entry of B_vals
, where each row contains
confidence intervals for one sub-sample replication.
If format = "long"
, the function returns a data.frame
with
one row for each CI_type
, each replication, and each entry of
B_vals
, where each row contains a single confidence interval for one
sub-sample replication.
If format = "wide-list"
, then the output will be structured as in
format = "wide"
but will be wrapped in an unnamed list, which makes
it easier to sore the output in a tibble, and will be assigned the class
"bootstrap_CIs"
.
vector of bootstrap replications of an estimator.
vector of estimated standard errors from each bootstrap replication.
numeric value of the estimate based on the original sample.
Required for CI_type = "normal"
, CI_type = "basic"
,
CI_type = "student"
, and CI_type = "bias-corrected"
.
numeric value of the estimated standard error based on the original
sample. Required for CI_type = "student"
.
vector of empirical influence values for the estimator. Required for CI_type = "BCa"
.
Character string or vector of character strings indicating
types of confidence intervals to calculate. Options are "normal"
,
"basic"
, "student"
, "percentile"
(the default), "bias-corrected"
, or "BCa"
.
numeric value between 0 and 1 for the desired coverage level,
with a default of 0.95
.
vector of sub-sample sizes for which to calculate confidence
intervals. Setting B_vals = length(boot_est)
(the default) will
return bootstrap confidence intervals calculated on the full set of
bootstrap replications. For B_vals < length(boot_est)
, confidence
intervals will be calculated after sub-sampling (without replacement) the
bootstrap replications.
integer value for the number of sub-sample confidence intervals
to generate when B_vals < length(boot_est)
, with a default of
reps = 1
.
character string controlling the format of the output. If
format = "wide"
(the default), then different types of confidence
intervals will be returned in separate columns. If format = "long"
,
then confidence intervals of different types will appear on different rows
of dataset. If format = "wide-list"
, then different types of
confidence intervals will be returned in separate columns and the result
will be wrapped in an unnamed list.
Single numeric value to which the random number generator seed
will be set. Default is NULL
, which does not set a seed.
Confidence intervals are calculated following the methods described
in Chapter 5 of Davison and Hinkley (1997). For basic non-parametric
bootstraps, the methods are nearly identical to the implementation in
boot.ci
from the boot
package.
Davison, A.C. and Hinkley, D.V. (1997). _Bootstrap Methods and Their Application_, Chapter 5. Cambridge University Press.
# generate t-distributed data
N <- 50
mu <- 2
nu <- 5
dat <- mu + rt(N, df = nu)
# create bootstrap replications
f <- \(x) {
c(
M = mean(x, trim = 0.1),
SE = sd(x) / sqrt(length(x))
)
}
booties <- replicate(399, {
sample(dat, replace = TRUE, size = N) |>
f()
})
res <- f(dat)
# calculate bootstrap CIs from full set of bootstrap replicates
bootstrap_CIs(
boot_est = booties[1,],
boot_se = booties[2,],
est = res[1],
se = res[2],
CI_type = c("normal","basic","student","percentile","bias-corrected"),
format = "long"
)
# Calculate bias-corrected-and-accelerated CIs
inf_vals <- res[1] - sapply(seq_along(dat), \(i) f(dat[-i])[1])
bootstrap_CIs(
boot_est = booties[1,],
est = res[1],
influence = inf_vals,
CI_type = c("percentile","bias-corrected","BCa"),
format = "long"
)
# calculate multiple bootstrap CIs using sub-sampling of replicates
bootstrap_CIs(
boot_est = booties[1,],
boot_se = booties[2,],
est = res[1],
se = res[2],
CI_type = c("normal","basic","student","percentile","bias-corrected"),
B_vals = 199,
reps = 4L,
format = "long"
)
# calculate multiple bootstrap CIs using sub-sampling of replicates,
# for each of several sub-sample sizes.
bootstrap_CIs(
boot_est = booties[1,],
boot_se = booties[2,],
est = res[1],
se = res[2],
CI_type = c("normal","basic","student","percentile"),
B_vals = c(49,99,199),
reps = 4L,
format = "long"
)
Run the code above in your browser using DataLab