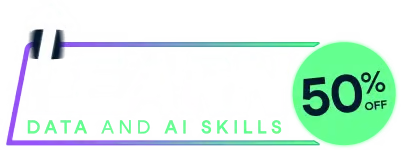
Last chance! 50% off unlimited learning
Sale ends in
# 'robject' converters
cvtCharacterFromJava(x, thisClassName)
cvtCharacterToJava(x, ...)
matchCharacterToJava(x, ...)
cvtIntegerFromJava(x, thisClassName)
cvtIntegerToJava(x, ...)
matchIntegerToJava(x, ...)
cvtLogicalFromJava(x, thisClassName)
cvtLogicalToJava(x, ...)
matchLogicalToJava(x, ...)
cvtNumericFromJava(x, thisClassName)
cvtNumericToJava(x, ...)
matchNumericToJava(x, ...)
cvtRawFromJava(x, thisClassName)
cvtRawToJava(x, ...)
matchRawToJava(x, ...)
cvtComplexFromJava(x, thisClassName)
cvtComplexToJava(x, ...)
matchComplexToJava(x, ...)
cvtVectorFromJava(x, thisClassName)
cvtVectorToJava(x, ...)
matchVectorToJava(x, ...)
cvtListFromJava(x, thisClassName)
cvtListToJava(x, ...)
matchListToJava(x, ...)
cvtArrayFromJava(x, thisClassName)
cvtArrayToJava(x, ...)
matchArrayToJava(x, ...)
cvtMatrixFromJava(x, thisClassName)
cvtMatrixToJava(x, ...)
matchMatrixToJava(x, ...)
cvtFactorFromJava(x, thisClassName)
cvtFactorToJava(x, ...)
matchFactorToJava(x, ...)
cvtDataFrameFromJava(x, thisClassName)
cvtDataFrameToJava(x, ...)
matchDataFrameToJava(x, ...)
cvtEnvFromJava(x, thisClassName)
cvtEnvToJava(x, ...)
matchEnvToJava(x, ...)
cvtUnknownFromJava(x, thisClassName)
cvtUnknownToJava(x, ...)
matchUnknownToJava(x, ...)
cvtFileReferencesFromJava(x, thisClassName)
cvtFileReferencesToJava(x, ...)
matchFileReferencesToJava(x, ...)
## 'javalib' converters
cvtCharacterToJava2(x, ...)
matchCharacterToJava2(x, ...)
cvtIntegerToJava2(x, ...)
matchIntegerToJava2(x, ...)
cvtLogicalToJava2(x, ...)
matchLogicalToJava2(x, ...)
cvtNumericToJava2(x, ...)
matchNumericToJava2(x, ...)
cvtRawToJava2(x, ...)
matchRawToJava2(x, ...)
cvtComplexFromJava2(x, thisClassName)
cvtComplexToJava2(x, ...)
matchComplexToJava2(x, ...)
cvtListToJava2(x, ...)
matchListToJava2(x, ...)
cvtFactorFromJava2(x, thisClassName)
cvtFactorToJava2(x, ...)
matchFactorToJava2(x, ...)
cvtDataFrameFromJava2(x, thisClassName)
cvtDataFrameToJava2(x, ...)
matchDataFrameToJava2(x, ...)
cvtCharArrayFromJava2(x, thisClassName)
cvtCharArrayToJava2(x, ...)
matchCharArrayToJava2(x, ...)
cvtIntegerArrayFromJava2(x, thisClassName)
cvtIntegerArrayToJava2(x, ...)
matchIntegerArrayToJava2(x, ...)
cvtNumericArrayFromJava2(x, thisClassName)
cvtNumericArrayToJava2(x, ...)
matchNumericArrayToJava2(x, ...)
cvtLogicalArrayFromJava2(x, thisClassName)
cvtLogicalArrayToJava2(x, ...)
matchLogicalArrayToJava2(x, ...)
cvtRawArrayFromJava2(x, thisClassName)
cvtRawArrayToJava2(x, ...)
matchRawArrayToJava2(x, ...)
cvtComplexArrayFromJava2(x, thisClassName)
cvtComplexArrayToJava2(x, ...)
matchComplexArrayToJava2(x, ...)
cvtCharMatrixFromJava2(x, thisClassName)
cvtCharMatrixToJava2(x, ...)
matchCharMatrixToJava2(x, ...)
cvtIntegerMatrixFromJava2(x, thisClassName)
cvtIntegerMatrixToJava2(x, ...)
matchIntegerMatrixToJava2(x, ...)
cvtNumericMatrixFromJava2(x, thisClassName)
cvtNumericMatrixToJava2(x, ...)
matchNumericMatrixToJava2(x, ...)
cvtLogicalMatrixFromJava2(x, thisClassName)
cvtLogicalMatrixToJava2(x, ...)
matchLogicalMatrixToJava2(x, ...)
cvtRawMatrixFromJava2(x, thisClassName)
cvtRawMatrixToJava2(x, ...)
matchRawMatrixToJava2(x, ...)
cvtComplexMatrixFromJava2(x, thisClassName)
cvtComplexMatrixToJava2(x, ...)
matchComplexMatrixToJava2(x, ...)
cvtEnvFromJava2(x, thisClassName)
cvtEnvToJava2(x, ...)
matchEnvToJava2(x, ...)
cvtFileReferencesFromJava2(x, thisClassName)
cvtFileReferencesToJava2(x, ...)
matchFileReferencesToJava2(x, ...)
cvtArrayFromJava
), or a R object.x
refers to.cvt*ToJava
functions return references to Java objects. The
cvt*FromJava
functions return instances of R
objects. match*
return TRUE
when the match criterion is
satisfied, FALSE
otherwise.
cvt*
functions take an instance of an object of one
language (R or Java) and convert it to an instance of the other
language. The match*
functions take an instance of an object, and return
a logical indicating whether the match criterion is satisified.
The convention used in RWebServices is to name the functions as
or
.
RWebServices
installs one of two types of converters. The
the function regAddonCvt
installs converters for the
‘robject’ model, where R objects are represented in a hierarchy
of Java objects that attempt to capture important attributes of the R
instances. The mapping is as follows:
R |
Java |
raw |
RRaw |
logical |
RLogical |
character |
RChar |
integer |
RInteger |
numeric |
RNumeric |
complex |
RComplex |
list RList factor RFactor data.frame RDataFrame environment REnvironment
array RArray matrix RMatrix
The ‘other’ converter is invoked when no other converter
matches. cvtUnknownToJava
creates an instance of the Java class
rservices.RUnknown
to hold the class
, length
, and
string representation (i.e., the result of print
) of the contents
of the R object. cvtUnknownFromJava
converts
rservices.RUnknown
instance to a R S4 instance if the original
R object is a S4 instance, otherwise the function returns a list with the
same class
and length
as the original R object. This is
likely to be very unsatisfactory.
The function regAddonCvt2
installs converters for the
‘javalib’ model, where R objects are represented by Java
primitive types where possible; NA
values are not
permitted. The mapping is as follows:
R |
Java |
raw |
byte[] |
logical |
boolean[] |
character |
String[] |
integer |
int[] |
numeric |
double[] |
complex |
RJComplex |
list Object[] factor RJFactor data.frame RJDataFrame environment java.util.HashMap
‘*’Array, RJ‘*’Array ‘*’Matrix, RJ‘*’Matrix
FileReferences RJFileReferences
The ‘*’Array and ‘*’Matrix and array entries represent
instances of the ArrayAndMatrix-class
, which provide
strong type information about the typeof
elements (raw,
logical, character, integer, numeric, complex) in arrays and
matricies.
setJavaFunctionConverter
,
regAddonCvt
,
regAddonCvt2